LitDev Extension API
LDShapes
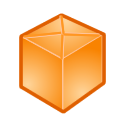
Shapes extension utilities.
AddAnimatedGif(imageName,repeat)
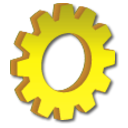
Creates an animated gif shape.
Do not add a very large number of these or performance may be degraded.
imageName The animated gif file (local or network) to load.
repeat Continuously repeat the animation "True" or "False".
returns The animated gif shape name.
AddAnimatedImage(imageName,repeat,countX,countY)
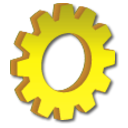
Creates an animation from a single image with multiple images on one layer.
Do not add a very large number of these or performance may be degraded.
imageName The image file (local or network) to load.
Can also be an ImageList image.
repeat Continuously repeat the animation "True" or "False".
countX The number of sub-images in the X direction.
countY The number of sub-images in the Y direction.
returns The animated shape name.
AddPolygon(points)
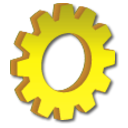
Creates a polygon shape.
points An array of coordinates for the polygon corners with the form points[i][1] = x, points[i][2] = y.
The number of points must be 3 or more.
returns The polygon shape name.
AddRegularPolygon(numPoint,radius)
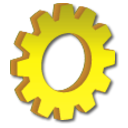
Creates a regular polygon shape.
numPoint The number of polygon sides.
radius The centre to corner distance.
returns The regular polygon shape name.
AddStar(numPoint,innerRadius,outerRadius)
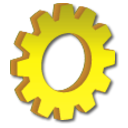
Creates a star shape.
Fun effects can be created with negative distances.
numPoint The number of star points.
innerRadius The centre to inner points' distance.
outerRadius The centre to outer points' distance.
returns The star shape name.
Angle(shapeName)
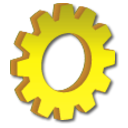
Get the shape's rotation angle.
shapeName The shape or control name.
returns The shape rotation in degrees.
AnimateOpacity(shapeName,interval,count)
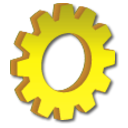
Set a shape to animate opacity, flash (fade out and in).
shapeName The shape or control to flash.
interval The interval in ms for a complete flash cycle.
A value of 0 will stop the flashing.
count The number of flashes.
A value of 0 will flash continuously.
AnimateRotation(shapeName,interval,count)
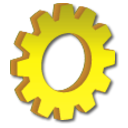
Set a shape to animate rotation (rotate continuously).
shapeName The shape or control to rotate.
interval The interval in ms for a complete 360 degree rotation.
A value of 0 will stop the rotation.
A value less than 0 will rotate anti-clockwise.
count The number of rotations.
A value of 0 will rotate continuously.
AnimateZoom(shapeName,interval,count,scaleX,scaleY)
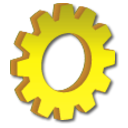
Set a shape to animate zooming (in and out).
shapeName The shape or control to zoom.
interval The interval in ms for a complete zoom cycle.
A value of 0 will stop the zooming.
count The number of zoom cycles.
A value of 0 will zoom continuously.
scaleX The X zoom scale factor.
scaleY The Y zoom scale factor.
AnimationCount(shapeName)
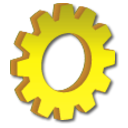
Get the number of images in an animated image.
shapeName The animated image shape name.
returns The number of images in the animated image.
AnimationInterval
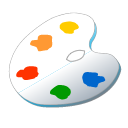
The update interval for animated images in ms (default 100).
A value of zero will pause all animated image updates, for example allowing AnimationSet to set images as required.
This is an internal timer that applies to all animated images that are not paused or completed a non-repeating cycle.
AnimationPause(shapeName)
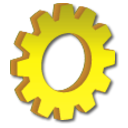
Pause an animated image.
Paused images can still be updated using AnimationSet
shapeName The animated image shape name.
AnimationResume(shapeName)
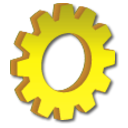
Resume a previously paused animated image.
shapeName The animated image shape name.
AnimationSet(shapeName,image)
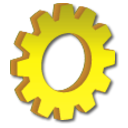
Reset animated image to a selected image.
shapeName The animated image shape name.
image The selected animated image number (indexed from 1).
BrushColour(shapeName,colour)
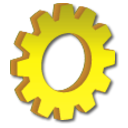
Set shape Brush colour.
shapeName The shape or control name.
colour The new brush colour.
BrushEllipse(brush,x,y,width,height)
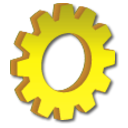
Draw an ellipse filled with a gradient of colours.
brush A previously created gradient or image brush (LDShapes.BrushGradient LDShapes.BrushImage).
x The x co-ordinate of the ellipse.
y The y co-ordinate of the ellipse.
width The width of the ellipse.
height The height of the ellipse.
returns None.
BrushGradient(colours,orientation)
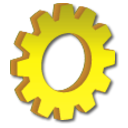
Create a linear or radial gradient brush.
colours An array of colours to apply to the shape in a gradient.
orientation The orientation for the gradient.
"H" horizontal.
"V" vertical.
"DU" diagonally up.
"DD" diagonally down.
"R" radial (default if "")
returns The gradient brush name.
BrushImage(imageName)
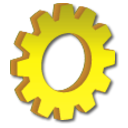
Create an image brush.
These brushes should work anywhere that BrushGradient can be used.
imageName The image to load to the brush.
Value returned from ImageList.LoadImage or local or network image file.
returns The image brush name.
BrushPolygon(brush,points)
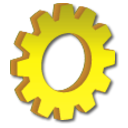
Draw a polygon filled with a gradient of colours.
brush A previously created gradient or image brush (LDShapes.BrushGradient LDShapes.BrushImage).
points An array of coordinates for the polygon corners with the form points[i][1] = x, points[i][2] = y.
The number of points must be 3 or more.
returns None.
BrushRectangle(brush,x,y,width,height)
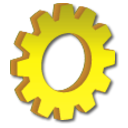
Draw a rectangle filled with a gradient of colours.
brush A previously created gradient or image brush (LDShapes.BrushGradient LDShapes.BrushImage).
x The x co-ordinate of the rectangle.
y The y co-ordinate of the rectangle.
width The width of the rectangle.
height The height of the rectangle.
returns None.
BrushRoundedRectangle(brush,x,y,width,height,radius)
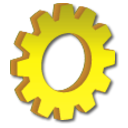
Draw a rounded rectangle filled with a gradient of colours.
brush A previously created gradient or image brush (LDShapes.BrushGradient LDShapes.BrushImage).
x The x co-ordinate of the rectangle.
y The y co-ordinate of the rectangle.
width The width of the rectangle.
height The height of the rectangle.
radius The radius of the rounded corners.
returns None.
BrushShape(shapeName,brush)
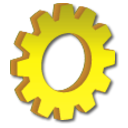
Set a shape Brush style as a gradient of colours.
shapeName The shape or control name.
brush A previously created gradient or image brush (LDShapes.BrushGradient LDShapes.BrushImage).
returns None.
BrushText(text,background,foreground)
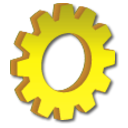
Create a text brush.
These brushes should work anywhere that BrushGradient can be used.
text The text to add to the brush.
The current GraphicsWindow font is used.
background The background colour.
foreground The foreground (pen) colour.
returns The text brush name.
Centre(shapeName,x,y)
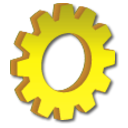
Centre the shape on a point, also works for zoomed shapes.
shapeName The shape or control name.
x The x coordinate of the centre.
y The y coordinate of the centre.
returns None.
FastMove(shapeName,x,y)
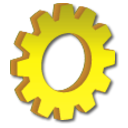
Moves the shape with the specified name to a new position.
This method is maximally optimised for speed.
shapeName The name of the shape to move.
x The x co-ordinate of the new position.
y The y co-ordinate of the new position.
Font(shapeName,family,size,bold,italic)
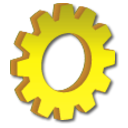
Set shape Font.
shapeName The shape or control name.
family The new font family name
See LDUtilities.FontList() for available font families.
size The new font size.
bold The new font bold state ("True" or "False").
italic The new font italic state ("True" or "False").
returns None.
GetAllShapes()
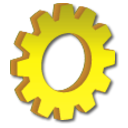
Get an array of all currently created shapes.
returns An array of shape names.
GetAllShapesAt(x,y)
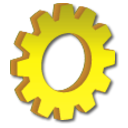
Get an array of all of the shapes (if any) at the specified coordinates.
The coordinates could be the mouse coordinates for example.
x The X coordinate
y The Y coordinate
returns An array of shape names or "False".
For multiple shapes, the returned array is ordered from top visual layer to bottom.
GetColour(shapeName)
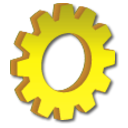
Get shape Brush and Pen colours.
shapeName The shape or control name.
returns A 3 element array
1) shape brush (or background) colour in hex format
2) shape opacity (0 to 100)
3) shape pen (or foreground) colour in hex format
GetLeft(shapeName)
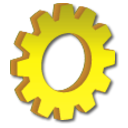
Get the left position of a shape (works for triangles, polygons and lines).
Also works for shapes while animating.
shapeName The shape or control name.
returns The x coordinate of the left edge of the shape.
GetOpacity(shapeName)
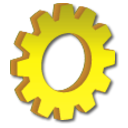
Gets the opacity of a shape.
shapeName The name of the shape.
returns The opacity of the object as a value between 0 and 100. 0 is completely transparent and 100 is completely opaque.
GetProperties(shapeName)
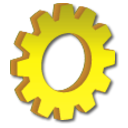
Get a list of shape properties. These are .Net UIElement properties.
shapeName The shape or control name.
returns An array of properties and their values.
GetProperty(shapeName,property)
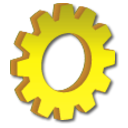
Get a shape property. This is a .Net UIElement property.
shapeName The shape or control name.
property The property name to get.
returns The value of the property.
GetTop(shapeName)
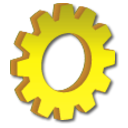
Get the top position of a shape (works for triangles, polygons and lines).
Also works for shapes while animating.
shapeName The shape or control name.
returns The y coordinate of the top edge of the shape.
Height(shapeName)
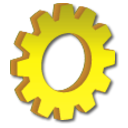
Get the shape's visible (including zoom) height.
shapeName The shape or control name.
returns The shape visible height.
LastEventShape
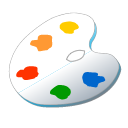
The last shape for which an event occured (ShapeEvent).
LastEventType
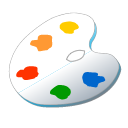
The last shape event type which an event occured (ShapeEvent). May be one of the following.
"MouseDown"
"MouseUp"
"MouseEnter"
"MouseLeave"
"GotFocus"
"LostFocus"
Move(shapeName,x,y)
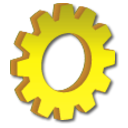
Moves a triangle or polygon top-left position.
This method also works for lines (Shapes.AddLine).
shapeName The shape name (a triangle, polygon or line shape).
x The X (left) coordinate for the triangle, polygon or line.
y The Y (top) coordinate for the triangle, polygon or line.
returns None.
MoveLine(shapeName,x1,y1,x2,y2)
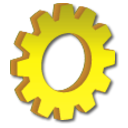
Moves a line shape.
shapeName The shape name (a line created with Shapes.AddLine).
x1 The first X coordinate to move the line to.
y1 The first Y coordinate to move the line to.
x2 The second X coordinate to move the line to.
y2 The second Y coordinate to move the line to.
returns None.
MovePolygon(shapeName,points)
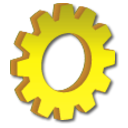
Moves a polygon shape.
shapeName The shape name (a polygon created with LDShapes.AddPolygon).
points An array of new coordinates for the polygon corners with the form points[i][1] = x, points[i][2] = y.
The number of points must be 3 or more and can change with each call.
returns None.
MoveTriangle(shapeName,x1,y1,x2,y2,x3,y3)
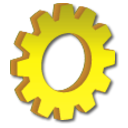
Moves a triangle shape.
shapeName The shape name (a triangle created with Shapes.AddTriangle).
x1 The first X coordinate to move the triangle to.
y1 The first Y coordinate to move the triangle to.
x2 The second X coordinate to move the triangle to.
y2 The second Y coordinate to move the triangle to.
x3 The third X coordinate to move the triangle to.
y3 The third Y coordinate to move the triangle to.
returns None.
Overlap(shape1,shape2)
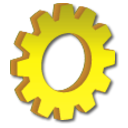
Checks for shape overlap (collision detection for any ellipse or rectangle shape types).
The first shape should be unZoomed and unRotated.
shape1 The first shape name.
shape2 The second shape name.
returns "True" or "False".
OverlapBox(shape1,shape2)
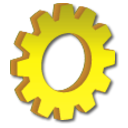
Checks for shape overlap of bounding boxes (collision detection).
shape1 The first shape name.
shape2 The second shape name.
returns "True" or "False".
OverlapCircle(shape1,shape2)
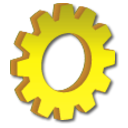
Checks for shape overlap of bounding circles (collision detection).
shape1 The first shape name.
shape2 The second shape name.
returns "True" or "False".
OverlapDetail
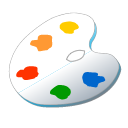
Following a call to an Overlap method, this property provides additional detail abut the intersection.
Result may be one of:
"Empty" - no intersection
"FullyContains" - shape1 is completely inside shape2
"Intersects" - overlap but neither is fully contained
"FullyInside" - shape2 is completely inside shape1
PenColour(shapeName,colour)
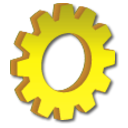
Set shape Pen colour.
shapeName The shape or control name.
colour The new pen colour.
returns None.
PenStyle(shapeName,dash,space)
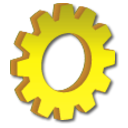
Set shape Pen style (dash, dot etc).
shapeName The shape name.
dash The dash length.
space The space length.
returns None.
PenWidth(shapeName,width)
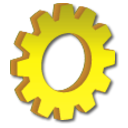
Set shape Pen width.
shapeName The shape or control name.
width The new pen width.
returns None.
RasteriseTurtleLines()
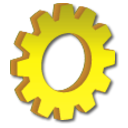
Rasterise all turtle trail lines.
When the number of turtle trails is large the program may slow due to the number of line shapes (trails) present.
This converts the turtle trails from line shapes to background drawings.
RemoveTurtleLines()
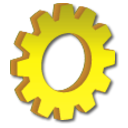
Remove all turtle trail lines.
ResetTurtle()
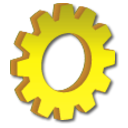
Reset the Turtle after a GraphicsWindow.Clear().
returns None.
ReSize(shapeName,width,height)
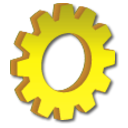
Resize shape width and height (an absolute version of zoom).
shapeName The shape or control name.
width The shape width.
height The shape height.
returns None.
RotateAbout(shapeName,x,y,angle)
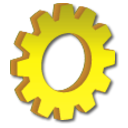
Rotate a shape about a point.
shapeName The shape name.
x The X coordinate to rotate the shape about.
y The Y coordinate to rotate the shape about.
angle The angle in degrees to rotate the shape.
returns None.
SetImage(shapeName,imageName)
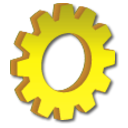
Set or change an image in a button or image shape.
shapeName The image or button name.
imageName The image to load.
Value returned from ImageList.LoadImage or local or network image file.
returns None.
SetProperty(shapeName,property,value)
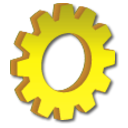
Set a shape property. This is a .Net UIElement property.
shapeName The shape or control name.
property The property name to set.
value The value to set the property to.
SetShapeEvent(shapeName)
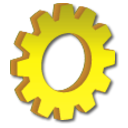
Register a shape to record mouse events: MouseDown, MouseUp, MouseEnter, MouseLeave, GotFocus and LostFocus.
shapeName The shape or control to add.
SetSize(shapeName,width,height)
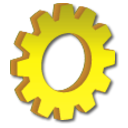
Reset the size of a shape as if it was created with the new size.
The position (top left point) is unchanged.
shapeName The shape or control name.
width The shape width.
height The shape height.
returns None.
SetTurtleImage(imageName,size)
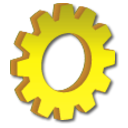
Set the turtle to an image.
imageName The image to load for the turtle.
Value returned from ImageList.LoadImage or local or network image file.
size The size to scale the turtle to (default turtle is 16).
ShapeEvent
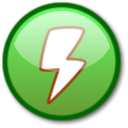
Event when a shape event occurs to a registered shape (SetShapeEvent)
Skew(shapeName,angleX,angleY)
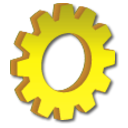
Skews the shape with the specified name by the specified angles.
shapeName The name of the shape to skew.
angleX The angle to skew the shape in the X direction.
angleY The angle to skew the shape in the Y direction.
returns None.
TextAlignment(shapeName,alignment)
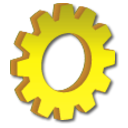
Set the alignment of text within a text shape
shapeName The shape or control name.
alignment The alignment, can be "Left", "Center", "Right" or "Justify"
TurtleSpeed
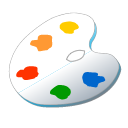
Allow turtle speeds greater than 10, a negative value is close to instant.
Width(shapeName)
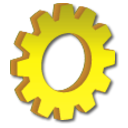
Get the shape's visible (including zoom) width.
shapeName The shape or control name.
returns The shape visible width.
ZIndex(shapeName,z_index)
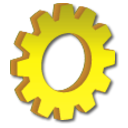
Set shape z index (layer position negative are background and positive are foreground - default 0).
shapeName The shape or control name.
z_index The z-index (zero, positive or negative interger).
returns None.
ZoomAll(scaleX,scaleY)
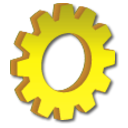
Zoom all shapes.
scaleX The x-axis zoom level.
scaleY The y-axis zoom level.